ゴール ユニットテストを実行できるようにする 1. ライブラリインストール 2. テスト用に関数作成 3. テストコード作成 4. package.jsonに実行コマンド追加 5. テストを実行する 6. importを省略する(任意) vitest.config.tsにtestに関する設定追加 tsconfig.jsonにも設定追加 カバレッジを表示する 1. 依存関係をインストール 2. package.jsonにコマンド追加 3. vite.config.tsにカバレッジに関する設定追加 4. gitignore追加 5. 実行
ゴール
- Vitestで
.ts
ファイルのユニットテストを作成できる - テストのカバレッジをUIで確認できるようにする
参考:Vitest
ユニットテストを実行できるようにする
1. ライブラリインストール
yarn add -D vitest
2. テスト用に関数作成
足し算するだけの関数を作成。
// app/services/calcService.ts
export function sum(a: number, b: number): number {
return a + b;
}
3. テストコード作成
1 + 2 = 3をテストする。
// test/services/calcService.test.ts
import { expect, test } from "vitest";
import { sum } from "~/services/calcService";
test("adds 1 + 2 equals 3", () => {
expect(sum(1, 2)).toBe(3);
});
4. package.jsonに実行コマンド追加
{
"scripts": {
"test": "vitest"
}
}
5. テストを実行する
1件のテストが成功していることが確認できる。
$ yarn run test
DEV v3.0.6 /Users/username/remix-sample
✓ test/services/calcService.test.ts (1 test) 3ms
✓ adds 1 + 2 equals 3
Test Files 1 passed (1)
Tests 1 passed (1)
Start at 20:09:12
Duration 613ms (transform 59ms, setup 0ms, collect 44ms, tests 3ms, environment 0ms, prepare 73ms)
PASS Waiting for file changes...
press h to show help, press q to quit
6. importを省略する(任意)
テストコードに都度 import { expect, test } from "vitest";
を書くのは煩わしいので、省略できるような設定をする。
もちろんこれは必須ではない。
参考:Vitest
vitest.config.tsにtestに関する設定追加
globals: true
を設定することにより、 vitest
がグローバルに提供されるようになる。
import { defineConfig } from 'vitest/config'
export default defineConfig({
test: {
globals: true,
},
})
tsconfig.jsonにも設定追加
{
"compilerOptions": {
"types": ["vitest/globals"]
}
}
これでvitestのimoprtをしなくても使えるようになる。
カバレッジを表示する
1. 依存関係をインストール
■カバレッジを表示するためのライブラリ
yarn add -D @vitest/coverage-v8
■テスト結果をUIで閲覧できるようにするライブラリ
yarn add -D @vitest/ui
2. package.jsonにコマンド追加
{
"scripts": {
"test": "vitest",
"test:coverage": "vitest run --coverage", // カバレッジを出力するため
"test:ui": "vitest --ui" // テスト結果のUIを表示するため
}
}
3. vite.config.tsにカバレッジに関する設定追加
export default defineConfig({
test: {
globals: true,
coverage: {
enabled: true, // trueで設定する
include: ["app/**"], // 対象をappディレクトリ以下にしておくと無駄がない
reporter: ["text", "html"], // text出力と、html形式での出力を指定
},
},
});
4. gitignore追加
カバレッジの結果は coverage/
ディレクトリに出力されるため、ignoreしておく。
# .gitignore
coverage/
5. 実行
■カバレッジ生成
reporterに text
の指定をしていると、コンソールからも確認することができる。
$ yarn run test:coverage
RUN v3.0.6 /Users/username/remix-sample
Coverage enabled with v8
✓ test/services/calcService.test.ts (1 test) 5ms
✓ adds 1 + 2 equals 3
Test Files 1 passed (1)
Tests 1 passed (1)
Start at 08:53:05
Duration 1.19s (transform 74ms, setup 0ms, collect 40ms, tests 5ms, environment 0ms, prepare 125ms)
% Coverage report from v8
-------------------|---------|----------|---------|---------|-------------------
File | % Stmts | % Branch | % Funcs | % Lines | Uncovered Line #s
-------------------|---------|----------|---------|---------|-------------------
All files | 1.04 | 66.66 | 66.66 | 1.04 |
app | 0 | 66.66 | 66.66 | 0 |
entry.client.tsx | 0 | 100 | 100 | 0 | 7-18
entry.server.tsx | 0 | 100 | 100 | 0 | 7-140
root.tsx | 0 | 0 | 0 | 0 | 1-45
app/routes | 0 | 50 | 50 | 0 |
_index.tsx | 0 | 100 | 100 | 0 | 3-138
sample.tsx | 0 | 0 | 0 | 0 | 1-7
app/services | 100 | 100 | 100 | 100 |
calcService.ts | 100 | 100 | 100 | 100 |
-------------------|---------|----------|---------|---------|-------------------
このエラーが気になった場合、以下の記事を参照することにより解消できる。
close timed out after 10000ms
Tests closed successfully but something prevents Vite server from exiting
You can try to identify the cause by enabling "hanging-process" reporter. See https://vitest.dev/config/#reporter
📄【Remix】Tests closed successfully but something prevents Vite server from exiting
■UIで確認
yarn run test:ui
このボタンからカバレッジレポートを視覚的に確認することができる。
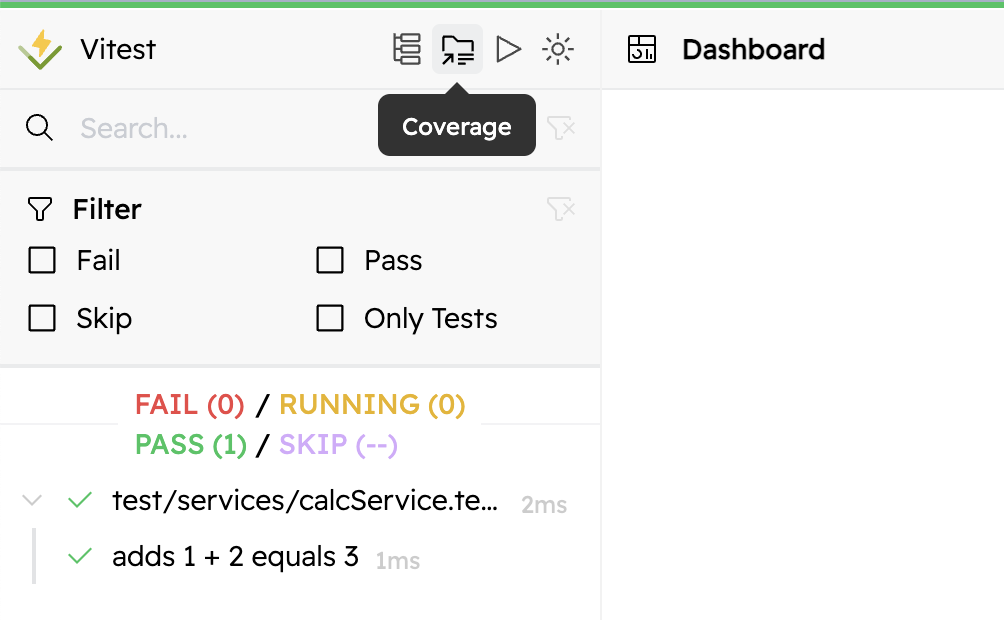
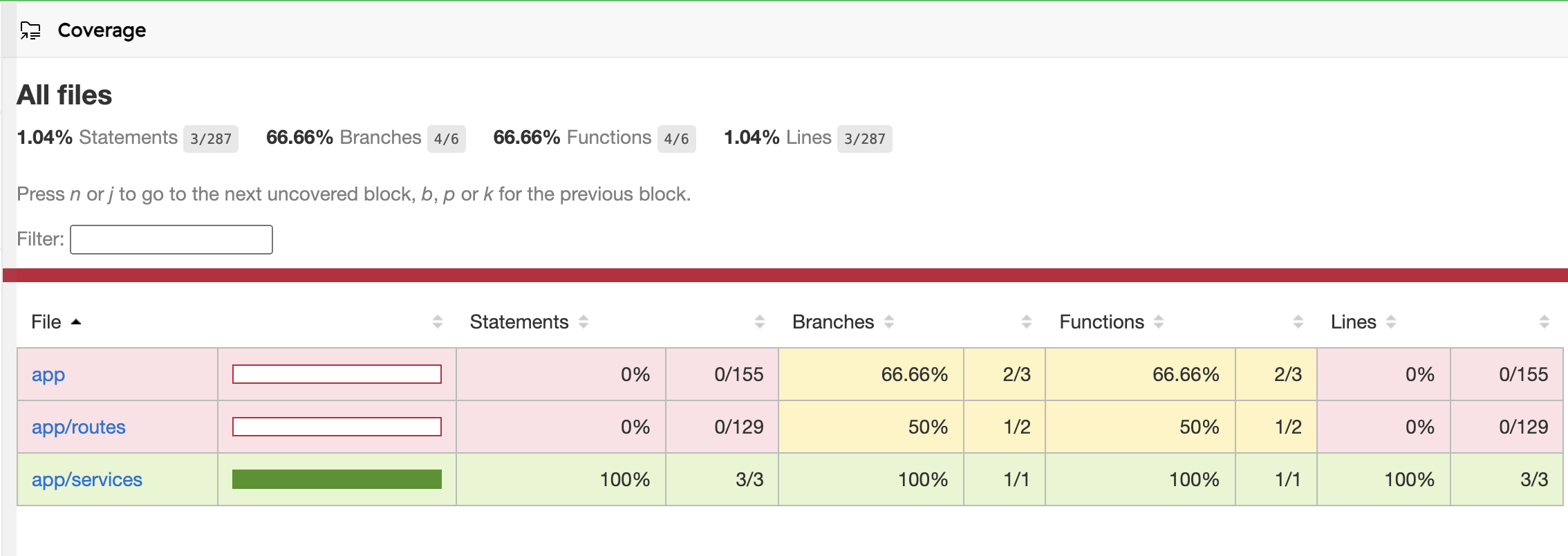